Did you know that 7 out of 10 developers with blogs also have a newsletter? Well, this isn't actually true. But, it is believable and I bet you were nodding along thinking yeah there are a lot of devs with newsletters.
The reason there are so many newsletters out there is because it's a fantastic way to cultivate an audience and to connect with your superfans. Inboxes aren’t like feeds, they aren’t affected by algorithms. Most people open their inbox and work down the list, so when someone signs up to your newsletter they are giving you direct access to them. With a newsletter your message isn't in the hands of the algorithm, it's up to the user to decide to open your email.
This means you can promote your content directly to those who want it, without the risk of being lost. It also means you can get direct feedback not from an egg profile but from real people as they can hit reply. I’ve used my newsletter to help me improve content before I've released it to a wider audience thanks to my amazing subscribers.
So if by now you don’t see the value in a newsletter I’m not sure I can convince you. However, if you see the value, it is pretty obvious why you want a newsletter. In fact, you probably already wanted one and that is why you are here. So enough waffling on, let’s look at how to start your own newsletter using Mailchimp and Nextjs.
Why Mailchimp?
When I was starting out with my newsletter I thought it was going to be huge. I imagined collecting hundreds of emails from the thousands of people that are already viewing my content. In reality, my newsletter at the time of writing this has ~500 subscribers. Not a bad amount of people, in fact, I’m quite proud of that number but it isn’t the 10,000 I thought I’d have.
So the first reason to use Mailchimp is that is completely free when you have less than 2000 subscribers. And, you'll probably have less than 2000 subscribers for a while.
The second reason to use Mailchimp is that it has great API support and lets you customize your newsletter form and sign-up process more so than some of its competitors.
With Mailchimp being the way to go at least early on, let’s look at how to set it up.
Setting up Mailchimp
Step one
You need to create a Mailchimp account.
Once you’ve signed up, you will need to get your API keys. You can follow the guide I just linked or if you're familiar with API keys you can click here to go directly to your keys.
If you went directly to your keys, then you need to click the "Create A Key" button like in the image below to get your API keys. Make sure you give your key a descriptive name so you know what it is for, something like "blog-newsletter-signup".
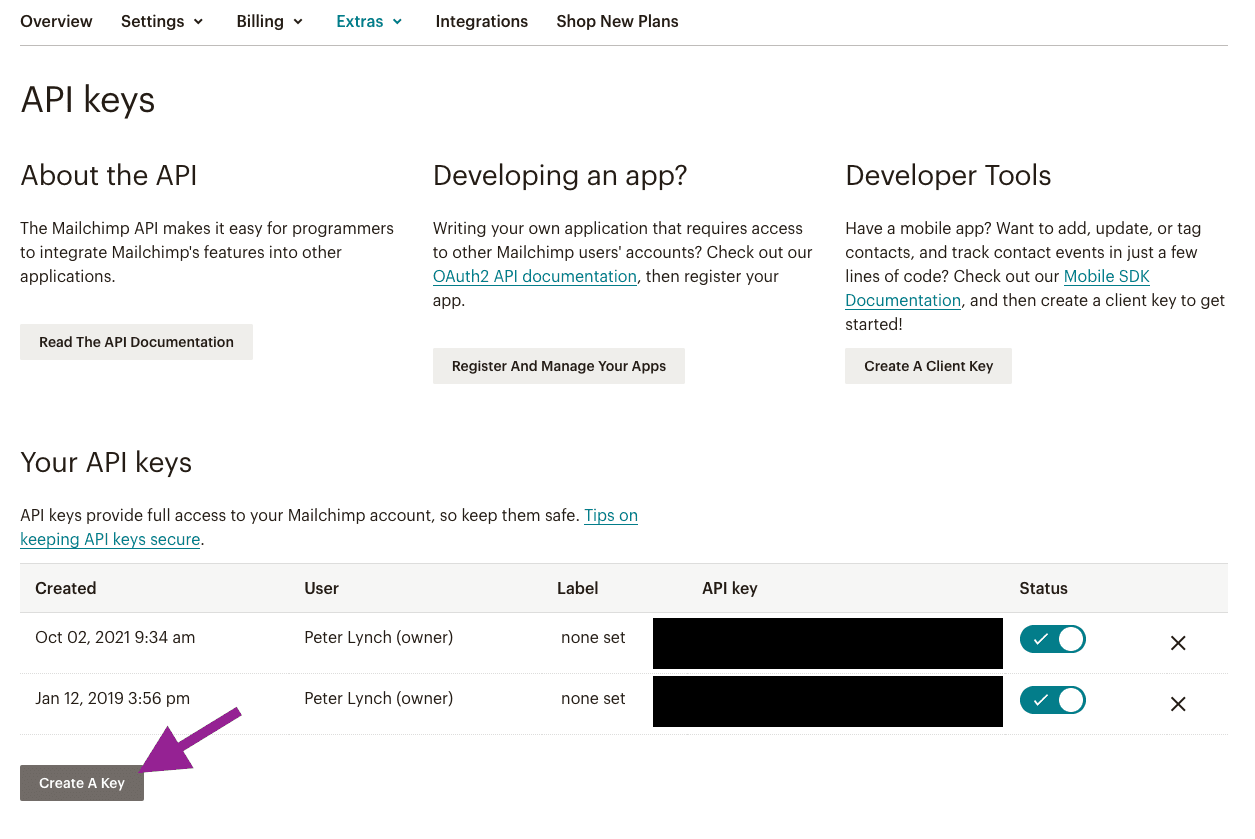
Once, you’ve created a key, copy it and put it somewhere safe so you can use it later.
Step two
Next, you need a way to add a user to your audience when they hit the "subscribe" button on your form. To do this you will need two things:
- The Audience ID for your mailing list.
- You get this by clicking, Audience → All Contacts → Settings → Audience Name & Defaults, then you will see your Audience ID → copy it add it to the same place you put your API key.
- Your API server region. To find this you need to be logged into your Mailchimp account, look at the URL in the browser and you’ll see something like
https://us20.admin.mailchimp.com/
theus20
is the server prefix we want.
With your account set up, your API key, Audience ID, and API server region you are ready to get into coding up your newsletter. Let’s start by creating some environment variables.
Environment Variables
To keep your audience id and API keys secure you want to create some environmental variables. Otherwise, someone could see your keys and Audience ID in the request data.
To create environmental variables you need to create a .env.local
file in the root directory of your project. This lets you access these values without hardcoding them to your form.
MAILCHIMP_API_KEY=xxxxxx-us20
MAILCHIMP_API_SERVER=us20
MAILCHIMP_AUDIENCE_ID=xxxxxx
The local file means you can test your newsletter signup locally, but you will also need to add them to your production environment. I use Vercel with Nextjs (it’s the best), using Vercel you add your environment variables like this. Other hosting providers will have their own methods, just google something like "add environmental variables host 'provider name'".
NaviListenComp-placeholder Keep your secrets
- Don’t forget to add your
.env.local
to your.gitignore
file. Otherwise when you commit and push up your changes these will appear on GitHub.
Once you put your values into your env.local file we can move on to making your form.
Creating a Newsletter Subscribe form
There are two parts to your Newsletter component, the first is the subscribeUser function which will send the request to your API. We haven’t created your API we will do that next.
The second part is the form itself. Have a look at the below snippet and we will break down how it works below.
import { useRef } from "react";
export default function NewsLetterSignUpForm() {
const inputRef = useRef(null);
const subscribeUser = async (e) => {
e.preventDefault();
// this is where your mailchimp request is made
const res = await fetch("/api/subscribeUser", {
body: JSON.stringify({
email: inputRef.current.value,
}),
headers: {
"Content-Type": "application/json",
},
method: "POST",
});
};
return (
<form onSubmit={subscribeUser}>
<label htmlFor='email-input' className='form__label'>
Your Best Email
</label>
<input
type='email'
id='email-input'
name='email'
placeholder='your best email'
ref={inputRef}
required
autoCapitalize='off'
autoCorrect='off'
/>
<button type='submit' value='' name='subscribe'>
Subscribe
</button>
</form>
);
}
Making Your Request
To add subscribers to your audience, you need to make a request to Mailchimp. You can achieve this by using Nextjs API routes.
You are already referencing your API in the form using the subscribeUser
function. You just need to create the API route to handle this.
First, create a new file subscribeUser
in the pages directory in a subfolder API. It should be pages/api/subscribeUser
.
import fetch from "isomorphic-unfetch";
export default async (req, res) => {
const { email } = req.body;
console.log({ email });
if (!email) {
return res.status(400).json({ error: "Email is required" });
}
try {
const AUDIENCE_ID = process.env.MAILCHIMP_AUDIENCE_ID;
const API_KEY = process.env.MAILCHIMP_API_KEY;
const DATACENTER = process.env.MAILCHIMP_API_SERVER;
const data = {
email_address: email,
status: "subscribed",
};
const response = await fetch(
`https://${DATACENTER}.api.mailchimp.com/3.0/lists/${AUDIENCE_ID}/members`,
{
body: JSON.stringify(data),
headers: {
Authorization: `apikey ${API_KEY}`,
"Content-Type": "application/json",
},
method: "POST",
}
);
if (response.status >= 400) {
return res.status(400).json({
error: `There was an error subscribing to the newsletter.
Hit me up peter@peterlunch.com and I'll add you the old fashioned way :(.`,
});
}
return res.status(201).json({ error: "" });
} catch (error) {
return res.status(500).json({ error: error.message || error.toString() });
}
};
Using Styled Components to Style the Form
Here is how I style my form if you are interested, sorry my blog source code is closed for the moment because of the way I write my posts.
Everything in the NewsletterSignUpForm
component above the return is the but the structure of my form is different.
export default function NewsLetterSignUpForm()
...
return (
<NewsletterMaxWidthWrapper>
<SignUpFormContainer>
<SignUpFormInnerContainer>
<SignUpFormContentContainer>
<NewsletterHeading>
A Web Development Newsletter That Helps You Create
</NewsletterHeading>
<NewsLetterAbout>
I'm learning heaps and having a blast doing it. Sign up for my
newsletter where I'll share the things I've made and how you can make
them too. Only real newsletters are going to last, all that other
bullshit is here today & gone tomorrow.
</NewsLetterAbout>
<Form onSubmit={subscribeUser}>
<EmailField>
<EmailInput
type="email"
id="email-input"
name="email"
placeholder="your best email"
ref={inputRef}
required
autoCapitalize="off"
autoCorrect="off"
/>
<Label htmlFor="email-input" className="form__label">
Your Best Email
</Label>
</EmailField>
<ButtonContainer>
<Subscribe type="submit" value="" name="subscribe">
<ButtonText>Subscribe</ButtonText>
<Icon></Icon>
</Subscribe>
</ButtonContainer>
</Form>
</SignUpFormContentContainer>
</SignUpFormInnerContainer>
</SignUpFormContainer>
</NewsletterMaxWidthWrapper>
)
I have a separate file NewsLetterSignUpFormStyles.js
where I write my styles. If you want access to them DM me on twitter, it's to much code to drop into this post.
That's how to make your own (if you use your own styles) Mailchimp Newsletter in Nextjs using styled-components. Now you can be one of the 7 developers out of 10 who have a newsletter congratulations.
You can save time and sign up for my newsletter below to learn other ways to improve your web pages.
Actually, you should probably check that it actually works by trying the newsletter sign-up form below. If it doesn’t work let me know on Twitter.